In this new Swift 2 tutorial you'll learn how to use the NSURLSession Swift function. You'll see different examples that illustrate how to define the different NSURLSession methods in various scenario, for downloading data or sending post requests.
Let's start this new tutorial by creating a new Swift iOS single view application.
For this tutorial we'll use iOS 9 as a deployment target and the examples are based on a server side script to get the data located on the server that does not have the https, so, for this reason, we have to edit the info.plist file, in order to allow this domain.
Edit the Info.plist to allow an http domain
Right click on the info.plist file, select open as, Source code. Add the lines of code that allow the http connection to this server.
<key>NSAppTransportSecurity</key> <dict> <key>NSExceptionDomains</key> <dict> <key>www.kaleidosblog.com</key> <dict> <key>NSTemporaryExceptionAllowsInsecureHTTPLoads</key> <true/> </dict> </dict> </dict>
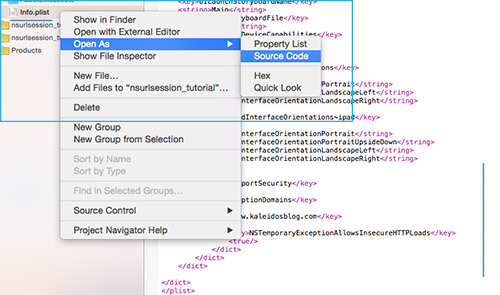
The NSURLSession is an asynchronous function and has three main principal methods:
- dataTaskWithUrl - dataTaskWithRequest
- downloadTaskWithUrl - downloadTaskWithRequest
- uploadTaskWithUrl - uploadTaskWithRequest
The dataTaskWithUrl is for normal data requests (when for example you'll have to ask to a web server for some data).
The downloadTaskWithUrl is for downloading files. It can resume the downloads for you and, as soon as the data is ready you'll have the temporary path in which the data is stored.
The uploadTaskWithUrl is for uploading data.
Server definition
The server in this example receives the data through a post request and simply replies it back. The post parameter has this format:
Post Request : 'data' > String
In this way we'll see if everythings works fine.
The url for the requests is located at this address:
https://www.kaleidosblog.com/tutorial/nsurlsession_tutorial.php
In swift I've defined a constant variable with this url, called url_to_request.
NSURLSession: dataTaskWithUrl example
Let's start this example with the dataTaskWithUrl function.
func data_request() { let url:NSURL = NSURL(string: url_to_request)! let session = NSURLSession.sharedSession() let request = NSMutableURLRequest(URL: url) request.HTTPMethod = "POST" request.cachePolicy = NSURLRequestCachePolicy.ReloadIgnoringCacheData let paramString = "data=Hello" request.HTTPBody = paramString.dataUsingEncoding(NSUTF8StringEncoding) let task = session.dataTaskWithRequest(request) { ( let data, let response, let error) in guard let _:NSData = data, let _:NSURLResponse = response where error == nil else { print("error") return } let dataString = NSString(data: data!, encoding: NSUTF8StringEncoding) print(dataString) } task.resume() }
The difference between dataTaskWithUrl and dataTaskWithRequest, as the name suggests, is that dataTaskWithUrl requires an URL as input, while the dataTaskWithRequest requires a NSMutableURLRequest variable. The difference between the URL and the NSMutableURLRequest is that the URL has to been initialized first with your requirments and nothing can change then, while with the NSMutableURLRequest you can define the variable and then you can set the behaviour like the cache policy, timeout, or the http method used. In this example the cache has been disabled in order to reload from the original source the request. The guard method let us check the response data.
NSURLSession: downloadTaskWithUrl example
func download_request() { let url:NSURL = NSURL(string: url_to_request)! let session = NSURLSession.sharedSession() let request = NSMutableURLRequest(URL: url) request.HTTPMethod = "POST" request.cachePolicy = NSURLRequestCachePolicy.ReloadIgnoringCacheData let paramString = "data=Hello" request.HTTPBody = paramString.dataUsingEncoding(NSUTF8StringEncoding) let task = session.downloadTaskWithRequest(request) { ( let location, let response, let error) in guard let _:NSURL = location, let _:NSURLResponse = response where error == nil else { print("error") return } let urlContents = try! NSString(contentsOfURL: location!, encoding: NSUTF8StringEncoding) guard let _:NSString = urlContents else { print("error") return } print(urlContents) } task.resume() }
The downloadTaskWithUrl stores in a temporary file the data you've received from the server. With the location variable you can access directly to this temporary file, or get the data from this file. This method is able to resume a suspended download.
NSURLSession: uploadTaskWithUrl example
func upload_request() { let url:NSURL = NSURL(string: url_to_request)! let session = NSURLSession.sharedSession() let request = NSMutableURLRequest(URL: url) request.HTTPMethod = "POST" request.cachePolicy = NSURLRequestCachePolicy.ReloadIgnoringCacheData let data = "data=Hi".dataUsingEncoding(NSUTF8StringEncoding) let task = session.uploadTaskWithRequest(request, fromData: data, completionHandler: {(data,response,error) in guard let _:NSData = data, let _:NSURLResponse = response where error == nil else { print("error") return } let dataString = NSString(data: data!, encoding: NSUTF8StringEncoding) print(dataString) } ); task.resume() }
This method is able to upload data to a server, by directly putting the data inside the formData variable.
Download the example
UPDATE: Download the swift 3 project example
Leave a comment